两个队列实现栈
栈和队列相似,都是特殊的线性表。
但在数据的运算方法与队列不同,栈为先进后出。队列是先进先出。
算法思路:
先初始化栈:初始化两个循环队列。
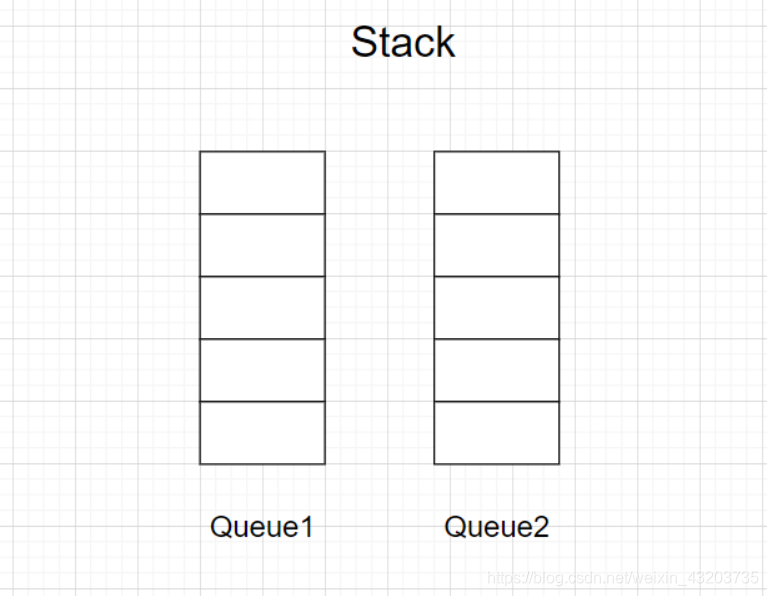
先存入数据:按照存入队列的方式将数据存入栈中。
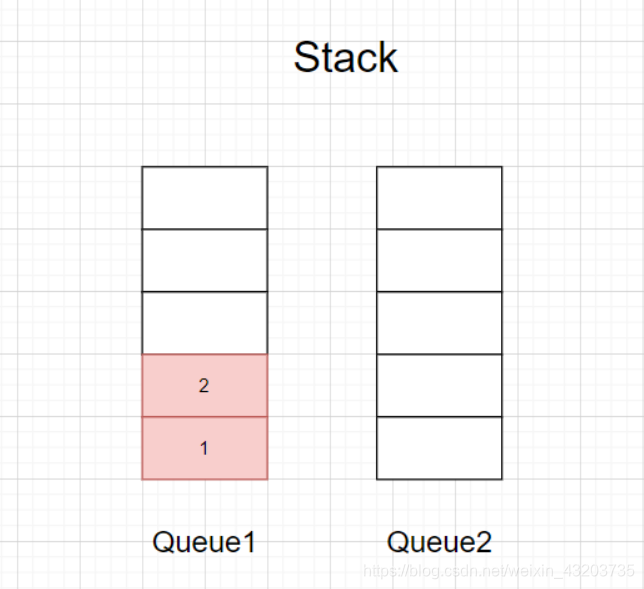
现在取出一个数据:我们将Queue1的数据依次存入Queue2中,直到Queue1只剩一个数据,然后将最后一个数据出队列。
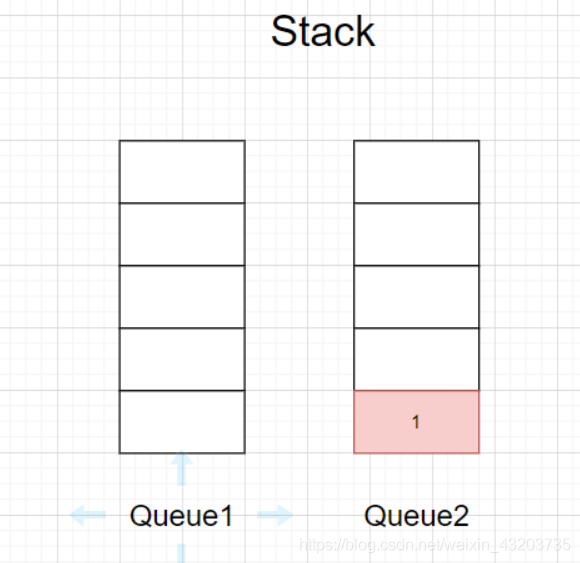
再加入数据:在已有数据的队列中添加数据。
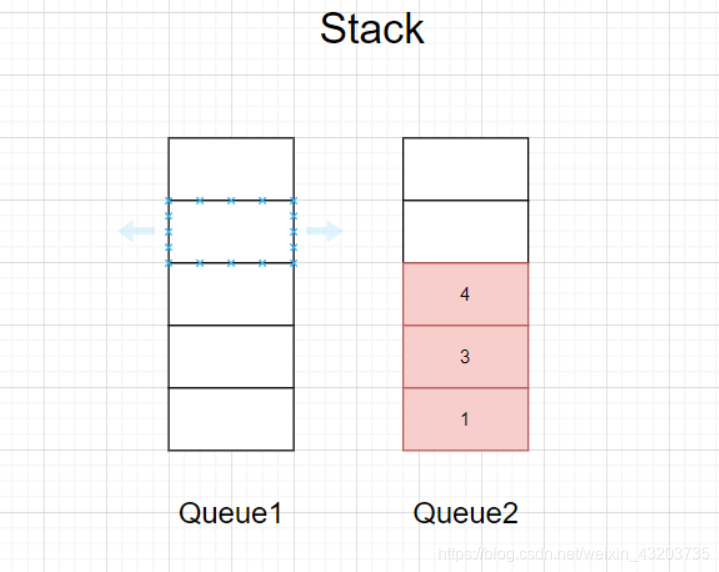
取出数据:同样,将Queue2的数据依次存入Queue1中,直到Queue2还剩最后一个数据,然后将最后一个数据出队列。
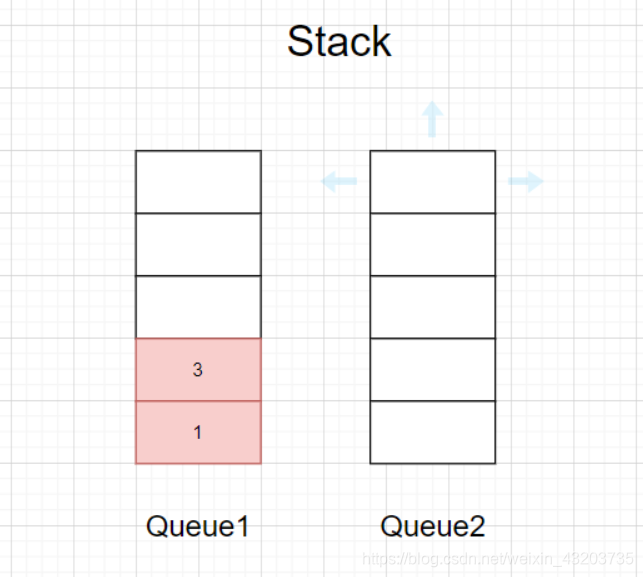
我们发现:
两个队列中至少有一个是空队列
在出栈时,将装有数据的队列循环到另一个空队列中,当数据只有一个时,这个数据就是需要出栈的,然后将这个数据出队列。
在进栈时,将数据添加进已有数据的队列中,如果两个都没有,默认存入Queue1。
代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65
| class StackByQueue{ ArrayCircularQueue Queue1; ArrayCircularQueue Queue2;
public StackByQueue(){ Queue1 = new ArrayCircularQueue(5); Queue2 = new ArrayCircularQueue(5); }
public boolean push(int i){ if(Queue1.isEmpty() && Queue1.isEmpty()){ return Queue1.AddQueue(i); }
if(!Queue1.isEmpty()){ return Queue1.AddQueue(i); }else{ return Queue2.AddQueue(i); } }
public Integer pop(){ if(Queue2.isEmpty() && Queue1.isEmpty()){ throw new RuntimeException("队列为空"); }
if (!Queue1.isEmpty() && Queue2.isEmpty() ){ while(Queue1.Size() > 1){ Queue2.AddQueue(Queue1.GetQueue()); } return Queue1.GetQueue(); } if (!Queue2.isEmpty() && Queue1.isEmpty() ){ while(Queue2.Size() > 1){ Queue1.AddQueue(Queue2.GetQueue()); } return Queue2.GetQueue(); }
return null; }
public void ShowStack() {
if(!Queue1.isEmpty()){ System.out.print("Queue1: "); Queue1.ShowQueue(); }else{ System.out.print("Queue2: "); Queue2.ShowQueue(); } }
public int Size(){ return Math.max(Queue1.Size(),Queue2.Size()); } }
|
测试:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| public static void main(String[] args) { StackByQueue stackByQueue = new StackByQueue();
stackByQueue.push(1); stackByQueue.push(2); stackByQueue.push(3); stackByQueue.push(4); stackByQueue.ShowStack();
System.out.println(stackByQueue.pop()); stackByQueue.ShowStack();
System.out.println(stackByQueue.pop());
System.out.println(stackByQueue.Queue1.isEmpty());
System.out.println(stackByQueue.Queue2.isEmpty());
stackByQueue.ShowStack();
System.out.println(stackByQueue.Size());
}
|